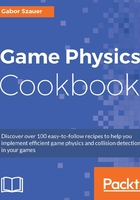
Translation
Translation is stored as a three-dimensional vector inside a 4 X 4 matrix. The translation component of the matrix describes how much to move an object on each axis. Because we decided to use Row Major matrices, translation is stored in elements 41, 42, and 43 of the matrix:

Getting Ready
We're going to implement three functions: one to retrieve the translation already stored inside a 4 X 4 matrix, one to return a translation matrix given x, y, and z components, and one to return a translation matrix given the same x, y, and z components packed inside a vec3
. When building any type of matrix, we start with the identity matrix and modify elements. We do this because the identity matrix has no effect on multiplication. The unused elements of a translation matrix should not affect rotation or scale; therefore we leave the first three rows the same as the identity matrix.
How to do it…
Follow these steps to set and retrieve the translation of a matrix:
- Add the declaration for all of the translation functions to
matrices.h
:mat4 Translation(float x, float y, float z); mat4 Translation(const vec3& pos); vec3 GetTranslation(const mat4& mat);
- Implement the functions that create 4 X 4 matrices in
matrices.cpp
. Because this matrix has no rotation, we start with the identity matrix and fill in only the elements related to translation:mat4 Translation(float x, float y, float z) { return mat4( 1.0f, 0.0f, 0.0f, 0.0f, 0.0f, 1.0f, 0.0f, 0.0f, 0.0f, 0.0f, 1.0f, 0.0f, x, y, z, 1.0f ); } mat4 Translation(const vec3& pos) { return mat4( 1.0f, 0.0f, 0.0f, 0.0f, 0.0f, 1.0f, 0.0f, 0.0f, 0.0f, 0.0f, 1.0f, 0.0f, pos.x,pos.y,pos.z,1.0f ); }
- Implement the function that retrieves the translation component of a 4 X 4 matrix in
matrices.cpp
:vec3 GetTranslation(const mat4& mat) { return vec3(mat._41, mat._42, mat._43); }
How it works…
Both Translation
functions return a new 4 X 4 matrix. This matrix is the identity matrix, with translation information stored in elements 41, 42, and 43. We start off with the identity matrix because we don't want the translation matrix to affect rotation or scale. The GetTranslation
function just needs to return elements 41, 42, and 43 packed into a vec3
structure.