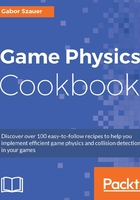
2D points
A point is the simplest two-dimensional primitive we can implement. It is infinitely small; it has x and y coordinates. A good way to think of a 2D point is like an alternate representation of a 2D vector. A vector points to somewhere in space; a point is where the vector points to:

Getting ready
Since this is the first geometry object we are creating, we also need to create a new header file, Geometry2D.h
. All future 2D geometry and intersection tests will be added to this file. Because a point has the same definition as a 2D vector, we're not going to create a new structure; instead we will redefine the vec2
struct as Point2D
.
How to do it…
Follow these steps to create a new header file in which we will define 2D geometry:
- Create a new C++ header file; call this file
Geometry2D.h
. - Add standard header guards to the file, and include
vectors.h
.#ifndef _H_2D_GEOMTRY_ #define _H_2D_GEOMETRY_ #include "vectors.h" #endif
- Because a point is practically the same thing as a 2D vector, we are not creating a new struct. Instead, we will redefine the
vec2
struct asPoint2D
:typedef vec2 Point2D;
How it works…
The typedef
specifier is a C++ language feature. It lets us create custom names for existing data types. When using a typedef
, the compiler will be aware that a Point2D
and vec2
are actually the same thing. This means we can use all the vector functions we've created with points! For example, we could find the distance between two points like this:
Point2D point1(1.0f, 3.0f); Point2D point2(7.0f, -3.0f); float distance = Magnitude(point1 - point2);