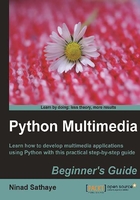
上QQ阅读APP看书,第一时间看更新
Time for action – pasting: mirror the smiley face!
Consider the example in earlier section where we cropped a region of an image. The cropped region contained a smiley face. Let's modify the original image so that it has a 'reflection' of the smiley face.
- If not already, download the file
Crop.png
from the Packt website. - Write this code by replacing the file path with appropriate file path on your system.
1 import Image 2 img = Image.open("C:\\images\\Crop.png") 3 # Define the elements of a 4-tuple that represents 4 # a bounding box ( region to be cropped) 5 left = 0 6 upper = 25 7 right = 180 8 lower = 210 9 bbox = (left, upper, right, lower) 10 # Crop the smiley face from the image 11 smiley = img.crop(bbox_1) 12 # Flip the image horizontally 13 smiley = smiley.transpose(Image.FLIP_TOP_BOTTOM) 14 # Define the box as a 2-tuple. 15 bbox_2 = (0, 210) 16 # Finally paste the 'smiley' on to the image. 17 img.paste(smiley, bbox_2) 18 img.save("C:\\images\\Pasted.png") 19 img.show()
- First we open an image and crop it to extract a region containing the smiley face. This was already done in section
Error: Reference source not found'Cropping'
. The only minor difference you will notice is the value of the tuple elementupper
. It is intentionally kept as 25 pixels from the top to make sure that the crop image has a size that can fit in the blank portion below the original smiley face. - The cropped image is then flipped horizontally with code on line 13.
- Now we define a box,
bbox_2
, for pasting the cropped smiley face back on to the original image. Where should it be pasted? We intend to make a 'reflection' of the original smiley face. So the coordinate of the top-right corner of the pasted image should be greater than or equal to the bottom y coordinate of the cropped region, indicated by 'lower
' variable (see line 8) . The bounding box is defined on line 15, as a2-tuple
representing the upper-left coordinates of the smiley. - Finally, on line 17, the paste operation is performed to paste the smiley on the original image. The resulting image is then saved with a different name.
- The original image and the output image after the paste operation is shown in the next illustration.
The illustration shows the comparison of original and resulting images after the paste operation.
What just happened?
Using a combination of Image.crop
and Image.paste
, we accomplished cropping a region, making some modifications, and then pasting the region back on the image.